This page shows Python examples of pygame.display. I'm guessing because pygame is hardware accelerated there are issues running the display on a remote desktop, also because tight vnc creates a separate desktop sessions it seems to me. I've tried initialized the pygame display with pygame.SWSURFACE but I still get the MIT-MAGIC-COOKIE-1 error. Closing the window We want our window to persist until the user chooses to closes it. To achieve this, we monitor user inputs (known as 'events') using pygame.event.get. This function returns a list of events which we can loop through and check to see whether any have the type QUIT.
Event
objects have a member variable (also called attributes or properties) named type
which tells us what kind of event the object represents. Pygame has a constant variable for each of possible types in the pygame.locals
modules. Line 9 checks if the Event object’s type
is equal to the constant QUIT
. Remember that since we used the from pygame.locals import *
form of the import
statement, we only have to type QUIT
instead of pygame.locals.QUIT
.
If the Event object is a quit event, then the pygame.quit()
and sys.exit()
functions are called. The pygame.quit()
function is sort of the opposite of the pygame.init()
function: it runs code that deactivates the Pygame library. Your programs should always call pygame.quit()
before they call sys.exit()
to terminate the program. Normally it doesn’t really matter since Python closes it when the program exits anyway. But there is a bug in IDLE that causes IDLE to hang if a Pygame program terminates before pygame.quit()
is called.
Since we have no if
statements that run code for other types of Event object, there is no event-handling code for when the user clicks the mouse, presses keyboard keys, or causes any other type of Event objects to be created. The user can do things to create these Event objects but it doesn’t change anything in the program because the program does not have any event-handling code for these types of Event objects. After the for
loop on line 8 is done handling all the Event objects that have been returned by pygame.event.get()
, the program execution continues to line 12.
Line 12 calls the pygame.display.update()
function, which draws the Surface object returned by pygame.display.set_mode()
to the screen (remember we stored this object in the DISPLAYSURF
variable). Since the Surface object hasn’t changed (for example, by some of the drawing functions that are explained later in this chapter), the same black image is redrawn to the screen each time pygame.display.update()
is called.
That is the entire program. After line 12 is done, the infinite while
loop starts again from the beginning. This program does nothing besides make a black window appear on the screen, constantly check for a QUIT
event, and then redraws the unchanged black window to the screen over and over again. Let’s learn how to make interesting things appear on this window instead of just blackness by learning about pixels, Surface objects, Color objects, Rect objects, and the Pygame drawing functions.
In Hello World we used the following line to create a Pygame window: screen = pygame.display.set_mode((640, 480), 0, 32)
The first parameter is the size of the window we want to create. A size of (640, 480) creates a small window that will fit comfortably on most desktops, but you can select a different size if you wish. Running in a window is great for debugging, but most games fill the entire screen with the action and don't have the usual borders and title bar. Full-screen mode is usually faster because your Pygame script doesn't have to cooperate with other windows on your desktop. To set full-screen mode, use the FULLSCREEN flag for the second parameter of set_mode:
screen = pygame.display.set_mode((640, 480), FULLSCREEN, 32)
■Caution If something goes wrong with your script in full-screen mode, it can sometimes be difficult to get back to your desktop. Therefore, it's best to test it in windowed mode first. You should also provide an alternative way to exit the script because the close button is not visible in full-screen mode.
Pygame Window Closing Automatically Download
When you go full screen, your video card will probably switch to a different video mode, which will change the width and height of the display, and potentially how many colors it can show at one time. Video cards only support a few combinations of size and number of colors, but Pygame will help you if you try to select a video mode that the card does not support directly. If the size of display you ask for isn't supported, Pygame will select the next size up and copy your display to the center of it, which may lead to black borders at the top and bottom of your display. To avoid these borders, select one of the standard resolutions that virtually all video cards support: (640, 480), (800, 600), or (1024, 768). To see exactly what resolutions your display supports, you can use pygame.display.list_modes(), which returns a list of tuples containing supported resolutions. Let's try this from the interactive interpreter:
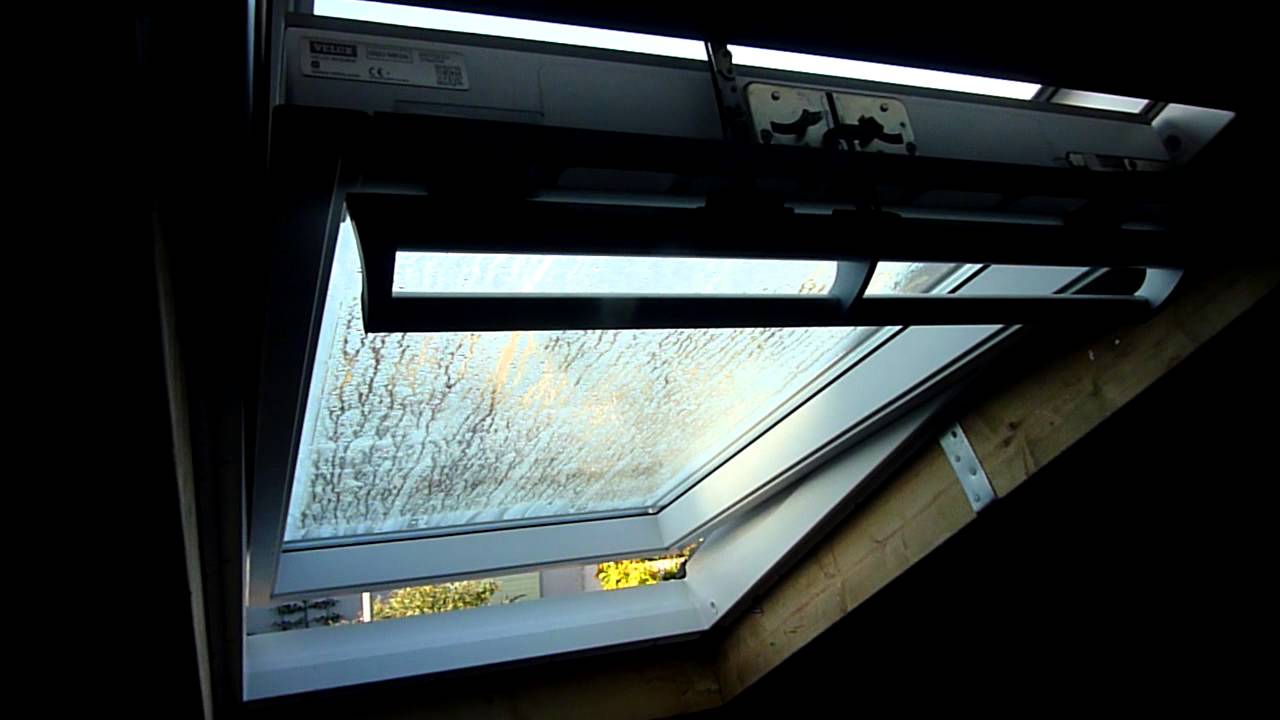
>>> import pygame >>> pygame.init() >>> pygame.display.list_modes()
[(800, 600), (1280, 1024), (1280, 960), (1280, 800), (1280, 768), (1280, 720), (1152, 864), (1088, 612), (1024, 768), (960, 600), (848, 480), (800, 600), (720, 576), (720, 480), (640, 480), (640, 400), (512, 384), (480, 360), (400, 300), (320, 240), (320, 200), (640, 480)]
If the video card can't give you the number of colors you asked for, Pygame will convert colors in the display surface automatically to fit (which may result in a slight drop in image quality).
Listing 3-4 is a short script that demonstrates going from windowed mode to full-screen mode. If you press the F key, the display will fill the entire screen (there may be a delay of a few seconds while this happens). Press F a second time, and the display will return to a window.
Listing 3-4. Full-Screen Example background_image_filename = 'sushiplate.jpg'
import pygame from pygame.locals import * from sys import exit pygame.init()
screen = pygame.display.set_mode((640, 480), 0, 32) background = pygame.image.load(background_image_filename).convert()

Fullscreen = False while True:
for event in pygame.event.get(): if event.type QUIT: exit()
Fullscreen = not Fullscreen if Fullscreen:
screen = pygame.display.set_mode((640 else:
screen = pygame.display.set_mode((640
screen.blit(background, (0,0)) pygame.display.update()
Pygame Window Closing Automatically Sign
Continue reading here: Additional Display Flags
Pygame Window Keeps Closing
Was this article helpful?